Say you want to design a ConservativePerson class.
1: import java.util.List;
2: class ConservativePerson{
3:
4:
5: private boolean isVirgin;
6: private boolean isMarried;
7: private List<String> children;
8:
9: ConservativePerson(boolean virgin, boolean married, List<String> children) {
10: this.isVirgin = virgin;
11: this.isMarried = married;
12: this.children = children;
13: }
14:
15: public boolean isVirgin() {
16: return isVirgin;
17: }
18: public boolean isMarried() {
19: return isMarried;
20: }
21:
22: public List<String> getChildren() {
23: return children;
24: }
25: }
As such it has some constrains.
- He must be married before he can be... well, not a virgin.
- He can't be a virgin before he can have children (as far as we know).
In the old days, which is basically all days until today..., you would probably define all kinds of modifiers methods for this class which will throw an exception in case of invariant invalidation such as NotMarriedException and VirginException. Not anymore.
Today we will do it by using the Wizard Design Pattern. We use a fluent interface style and utilize the power of a modern IDE to create a wizard-like feeling when building a ConservativePerson object. We know, we know, stop talking and show us the code... but before we will present the wizard code we will show you it usage so you will get a grasp of what we are talking about...
1: public class Main {
2: public static void main(String[] args) {
3: ConservativePersonWizardBuilder wizard = new ConservativePersonWizardBuilder();
4: ConservativePerson singlePerson = wizard.
5: createConservativePerson().
6: whichIsSingle().
7: getObject();
8: ConservativePerson familyManPerson = wizard.
9: createConservativePerson().
10: whichIsMarried().
11: andNotVirgin().
12: andHasChildNamed("Noa").
13: anotherChildNamed("Guy").
14: lastChildName("Alon").
15: getObject();
16: }
17:
18: }
Now, it may look just like an ordinarily fluent interface, but the cool thing here is that a method is available for calling only if the current object state allows it. This means you will not be able to call the method andNotVirgin if you haven't called the method whichIsMarried.
See the following set of screen shots:
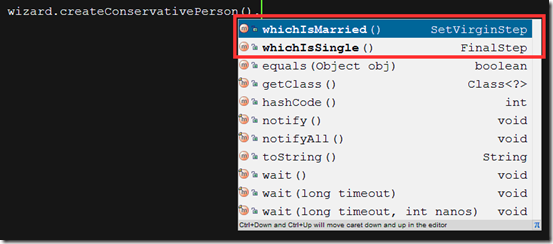
and after we state he is married we can:
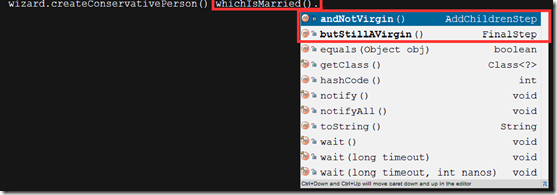
Here is the wizard code. I urge you to copy/paste it to your IDE and give it a try by building an object with it.
1: import java.util.ArrayList;
2: import java.util.List;
3:
4: public class ConservativePersonWizardBuilder {
5: private boolean isVirgin;
6: private boolean isMarried;
7: private List<String> children = new ArrayList<String>();
8:
9: public SetMarriedStep createConservativePerson(){
10: return new SetMarriedStep();
11: }
12:
13: class SetMarriedStep {
14: public SetVirginStep whichIsMarried(){
15: isMarried = true;
16: return new SetVirginStep();
17: }
18:
19: public FinalStep whichIsSingle(){
20: isMarried = false;
21: return new FinalStep();
22: }
23: }
24:
25: class SetVirginStep {
26: public AddChildrenStep andNotVirgin(){
27: isVirgin = false;
28: return new AddChildrenStep();
29: }
30: public FinalStep butStillAVirgin(){
31: isVirgin = true;
32: return new FinalStep();
33: }
34: }
35:
36: class FinalStep {
37: public ConservativePerson getObject(){
38: return new ConservativePerson(isVirgin, isMarried, children);
39: }
40: }
41:
42: class AddChildrenStep {
43: public AddChildrenStep andHasChildNamed(String childName) {
44: children.add(childName);
45: return new AddChildrenStep();
46: }
47: public AddChildrenStep anotherChildNamed(String childName) {
48: children.add(childName);
49: return new AddChildrenStep();
50: }
51: public FinalStep lastChildName(String childName){
52: children.add(childName);
53: return new FinalStep();
54: }
55: }
56: }
As you can see the wizard consists of several steps. Each step is represented by a dedicated inner class. Each step reveals the legal available operations by its methods. Each method will then return a new step according to the change it has made. This way an attempt to create an illegal object will be detected at compile time instead of runtime.
This pattern is actually being used in our production code. One example that comes to mind is the MediaJob class. This class describes a manipulation on some media files. In order to submit a job to the system, one has to create a MediaJob object. The problem is that this object has many parameters that could be assigned with contradicting values that create an illegal object state. By using the Wizard pattern, one can easily build a legal job without the need to know the entire (and complicated…) set of constrains.
That is all for now. Hope you'll give it a try..... We plan to write a more formal description of it (GOF style) in the near future.
Nice! I liked this one, need to try it on some time
ReplyDeleteBeen using it in my code for some time now - as for writing the code, it's not so fluent, as the name might suggest but the usage make the code much much more readable and easy to understand.
ReplyDeleteVery well done !
Thank you guys, happy to hear this is fruitful. Will keep on updating
DeleteIn the Java world, this is considered as just an extension to the Builder pattern and can be done more concisely with interfaces: http://stackoverflow.com/a/6613673
ReplyDeletewhat is the editor you are using :) , amazing post
ReplyDeletei love your blog, i have it in my rss reader and always like new things coming up from it.
ReplyDeleteYou ought to take part in a contest for one of the finest websites online. I will highly recommend this site!
ReplyDelete